There is a common error called “No QueryClient set, use QueryClientProvider to set one” of react-query that most newbies found complicated, so in this tutorial, I am going to show to how to fix this error.
Let solve this together!
A brief intro to React-Query
React Query is one of the best libraries for react developers, React Query is used for fetching, caching, synchronizing, and updating server state. React Query is hands down one of the best libraries for managing server state. It works amazingly well out-of-the-box, with zero-config, and can be customized to your liking as your application grows.
Fix: No QueryClient set, use QueryClientProvider to set one
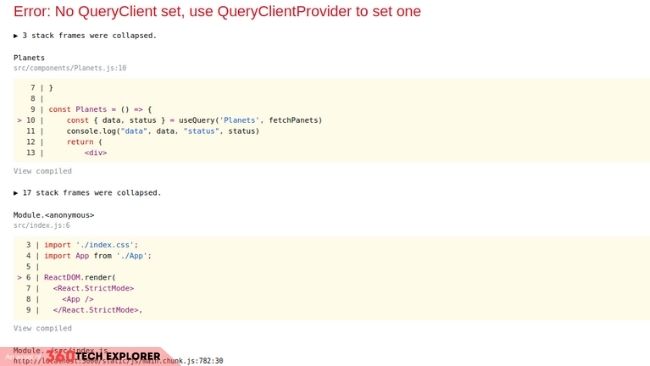
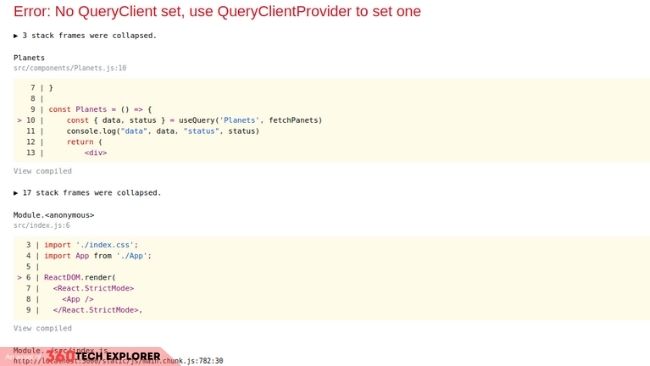
A PRO TIP: Read the error messages properly most of the time solution is available to an error message.
As the error suggests use QueryClientProvider and QueryClient. Here I divide this into 3 simple steps.
- Import the packages
import { QueryClient, QueryClientProvider, useQuery } from 'react-query'
- Make your components as usual
const Example = () => {
const { isLoading, error, data } = useQuery('repoData', () =>
fetch('https://api.github.com/repos/tannerlinsley/react-query').then(res =>
res.json()
)
)
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>{data.subscribers_count}</strong>{' '}
<strong>{data.stargazers_count}</strong>{' '}
<strong>{data.forks_count}</strong>
</div>
)
}
- Create the QueryClient and use it!
const queryClient = new QueryClient()
export default function Wraped(){
return(<QueryClientProvider client={queryClient} contextSharing={true}>
<Example/>
</QueryClientProvider>
);
}
Here docs,
QueryClientProvider
Use the QueryClientProvider component to connect and provide a QueryClient to your application
client: QueryClient
Required
the QueryClient instance to provide
contextSharing: boolean
defaults to false
Set this to true to enable context sharing, which will share the first and at least one instance of the context across the window to ensure that if React Query is used across different bundles or micro frontends they will all use the same instance of context, regardless of module scoping.
FINAL CODE
import { QueryClient, QueryClientProvider, useQuery } from 'react-query'
const queryClient = new QueryClient()
const Example = () => {
const { isLoading, error, data } = useQuery('repoData', () =>
fetch('https://api.github.com/repos/tannerlinsley/react-query').then(res =>
res.json()
)
)
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>{data.subscribers_count}</strong>{' '}
<strong>{data.stargazers_count}</strong>{' '}
<strong>{data.forks_count}</strong>
</div>
)
}
export default function Wraped(){
return(<QueryClientProvider client={queryClient} contextSharing={true}>
<Example/>
</QueryClientProvider>
);
}
What’s next?
You ready to start your application as usual. Feel free to ask anything in the comments below!