Welcome to this tutorial on how to get elements in array after a specific index in JavaScript! In this tutorial, we will be using the slice() method to extract the desired elements from an array. We will also look at its Algorithm, Visual Representation, and Implementation. By the end of this tutorial, you will have a good understanding of how to extract elements from an array in JavaScript.
There are multiple methods to get all elements in an array after a specific index in JavaScript.
Using slice() method in Javascript
You can use the slice() method. This method returns a new array that includes all elements from the start index to the end of the original array.
const index = 2 // This is your specific index
const array = [1, 2, 3, 4, 5];
const slicedArray = array.slice(index);
// slicedArray is [3, 4, 5]
In the example above, the slice() method is called on the array with an index of 2 as the argument. This returns a new array that includes all elements from index 2 to the end of the array, which is [3, 4, 5].
Here I want to let you know that you can also specify the last index of the array.
const array = [1, 2, 3, 4, 5];
const slicedArray = array.slice(2, 4);
// slicedArray is [3, 4]
4 as the second argument. This returns a new array that includes all elements from index 2 up to but not including index 4, which is [3, 4].
Note: that the slice() method does not modify the original array. It creates a new array with the desired elements. Read more.
Algorithm
Here is the algorithm for the slice() method:
- Check if the slice() method is being called on an array-like object or a true array.
- If the object is array-like, convert it to a true array.
- If the start index is not a number, set it to 0.
- If the end index is not a number, set it to the length of the array.
- If the start index is greater than the end index, return an empty array.
- Initialize a new empty array called sliced.
- Loop through the array from the start index to the end index.
- For each element in the loop, add it to the sliced array.
- Return the sliced array.
Visual Representation
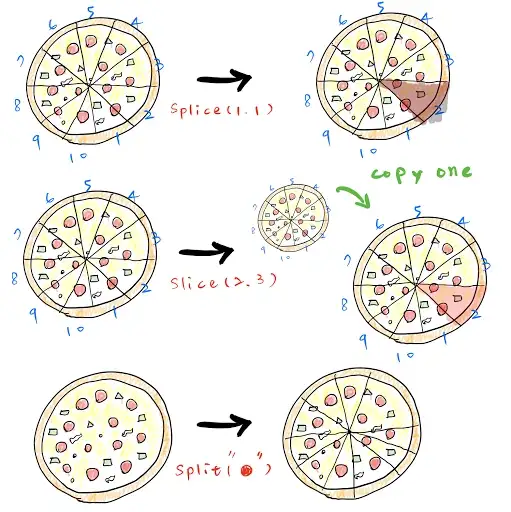
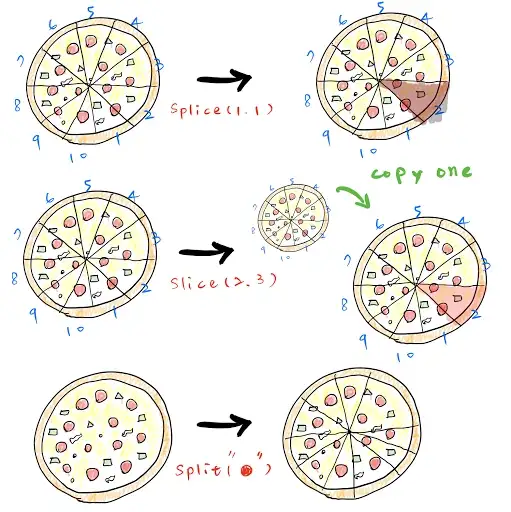
Implementation
Here is an implementation of the slice() method in JavaScript:
function slice(array, startIndex, endIndex) {
// Check if the slice() method is being called on an array-like object or a true array
if (!Array.isArray(array)) {
// If the object is array-like, convert it to a true array
array = Array.prototype.slice.call(array);
}
// If the start index is not a number, set it to 0
if (typeof startIndex !== 'number') {
startIndex = 0;
}
// If the end index is not a number, set it to the length of the array
if (typeof endIndex !== 'number') {
endIndex = array.length;
}
// If the start index is greater than the end index, return an empty array
if (startIndex > endIndex) {
return [];
}
// Initialize a new empty array called sliced
let sliced = [];
// Loop through the array from the start index to the end index
for (let i = startIndex; i < endIndex; i++) {
// For each element in the loop, add it to the sliced array
sliced.push(array[i]);
}
// Return the sliced array
return sliced;
}
Extra Knowlage
One thing that you may not know about the slice() method in JavaScript is that it can be used to shallow-copy elements from an array or array-like object into a new array.
For example, consider the following code:
const array = [1, 2, 3, 4, 5];
const copiedArray = array.slice();
// copiedArray is [1, 2, 3, 4, 5]
Ok, that is all I think is relevant information to your question, if you feel something is missing or if you want to ask something to me, Post a comment.