“ReferenceError: prompt is not defined” is a very common Javascript error in which you end up falling. This error simply means that the prompt function is not available (or you can say not defined) in your environment.
In this document, we will give you a piece of proper information about why this error occurred and how you can fix it.
What is a prompt() method in Javascript?
Javascript’s ‘prompt()’ method is defined inside ‘window’ object of Brower Environment which allows you to take input from the user through a little pop-up window.
When you use the ‘prompt()’ method in your code, a dialog box with a message (optional) and a text input field will appear on the screen. In this field, the user can enter their responses and then select “OK” or “Cancel.” The value they supplied will be returned as a string if they click “OK.” The method will return “null” if they choose to cancel.
For example, you can use the ‘prompt()’ method as follows to ask the user their name:
let name = prompt("What is your name?");
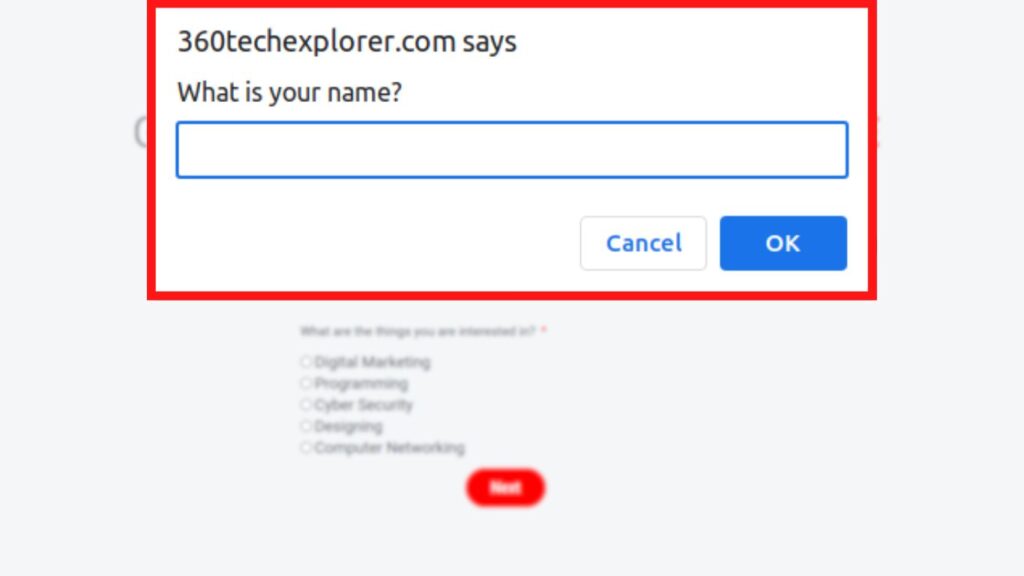
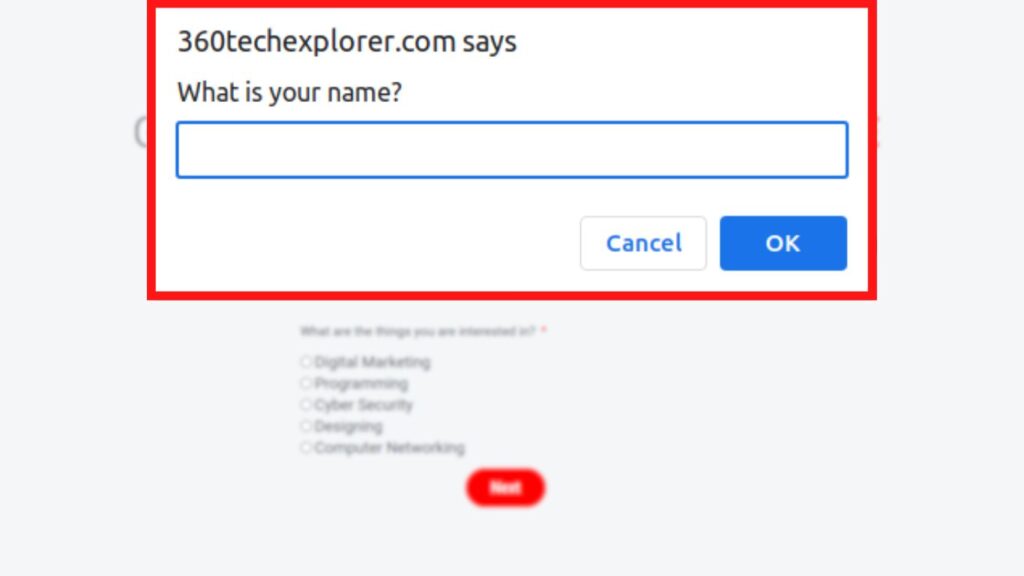
This will show a pop-up window with the question “What is your name?” and an input form. The user can fill out the section with their name and press “OK.” The variable ‘name’ will be updated with the value they entered.
Fix “ReferenceError: prompt is not defined”
“ReferenceError: prompt is not defined” occurs when you try to use the prompt method in a JavaScript environment that does not support it, such as a Node.js environment or a non-browser environment.
Tip: If you are trying to use the prompt function in a browser environment, make sure that you are using it in a script that is executed in the context of a web page. For example, you could add your JavaScript code to an HTML file and open it in a web browser.
If you are using a prompt in a non-browser environment, such as Node.js, you will need to use a different method for accepting user input. Here is the solution.
One option is to use ‘prompt-sync‘
First of all, install the package:
npm i prompt-sync
Here is an example of how to use prompt-sync to prompt the user for input:
const prompt = require("prompt-sync")({sigint:true})
const name = prompt('What is your name? ');
console.log("Hello " + name);
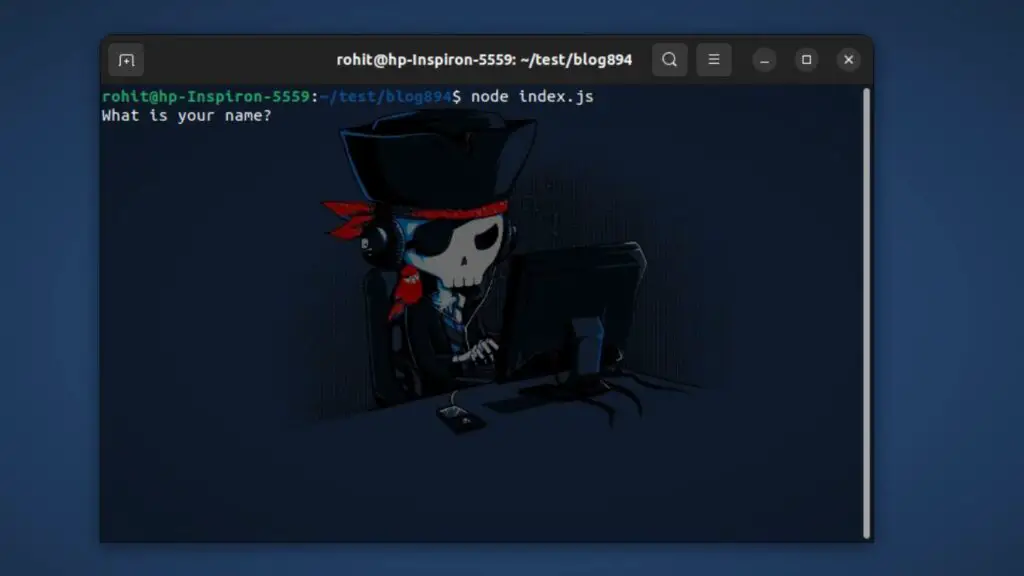
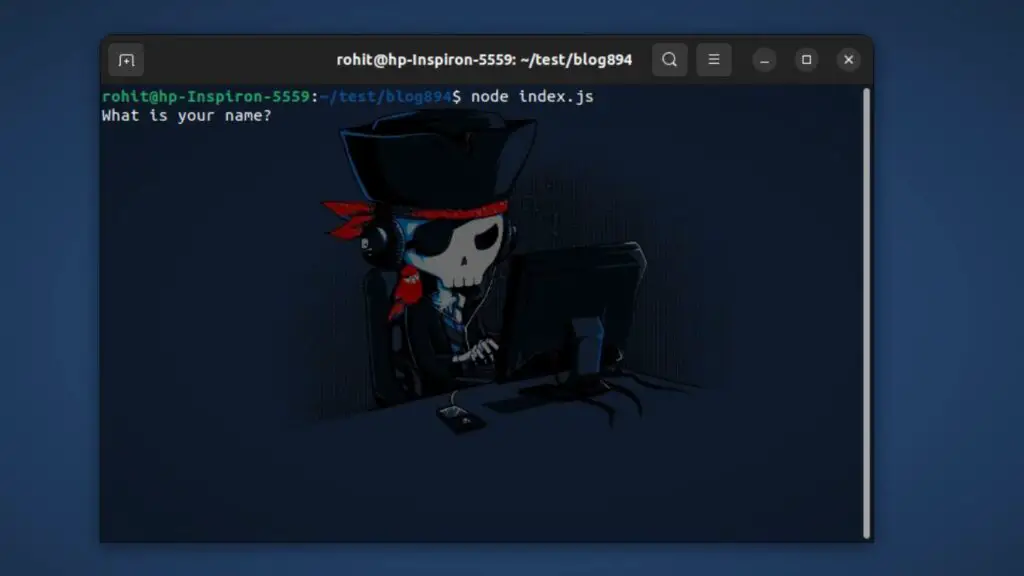
In this code, we use the prompt-sync package to create a synchronous input prompt for the user to enter their name. The input value is stored in the name variable, and then we log a greeting message to the console.
Another option is to use the readline module to read input from the command line. Here’s an example:
const readline = require('readline').createInterface({
input: process.stdin,
output: process.stdout
});
readline.question('Enter your name: ', (name) => {
console.log(Hello, ${name}!);
readline.close();
});
This code creates a readline interface and uses it to prompt the user to enter their name. When the user enters their name and presses Enter, the code logs a greeting message to the console.
Other huntable packages are mentioned below:
inquirer: This is a popular package for creating command-line interfaces with prompts and interactive user input. It provides a wide variety of input types and customization options.
enquirer: This is another package that provides a flexible and customizable prompt system for Node.js. It supports a wide variety of input types and validation options.
prompt: This package is similar to inquirer, but it is a bit simpler and provides fewer customization options. It supports a variety of input types and provides a fluent API for building prompts.
cli-interact: This is a lightweight package that provides a simple API for prompting the user for input. It supports basic input types like text, numbers, and passwords.
Fix “ReferenceError: prompt is not defined” in Brower Environment
- Check if you have any browser extensions or plugins installed that might be blocking the prompt function. Disable them temporarily and see if that resolves the issue.
- Try using a different browser or updating your current browser to the latest version.
- Make sure that your code is not in a frame or iframe, as this can cause issues with the prompt function.
- Check your browser’s security settings to make sure that JavaScript is enabled and that there are no settings blocking the use of the prompt function.
- If you are using a Content Security Policy (CSP) on your website, make sure that it allows the use of the unsafe-eval directive for scripts. The prompt() function may require this directive to be enabled in some cases.
- Some browsers block the prompt() function by default on websites because it can be used to create phishing attacks or other malicious behavior so make sure that you are accessing your script from a secure context, such as using HTTPS instead of HTTP.
Alternatively, you can use a different user input method instead of prompt(), such as a form input field or a modal dialog, depending on the requirements of your application.
Calculation
In conclusion, the JavaScript ‘prompt()’ method is a helpful function that lets you take user input through a pop-up window. The readline module in Node.js or other packages like inquirer, enquirer, prompt, cli-interact, and stdinput are other alternatives that developers can use to receive user input. By employing these options, programmers may guarantee that their apps can receive user input and function properly in a variety of settings.
I hope your issue is solved now. If it is still not solved please do let me know in the comments.