What is modular programming?
Modular programming or modular architecture is a way to distribute large software applications code into small pieces of code for better maintainability and sustainability. In this approach, the software is divided into multiple modules and each module performs a certain task of software application.
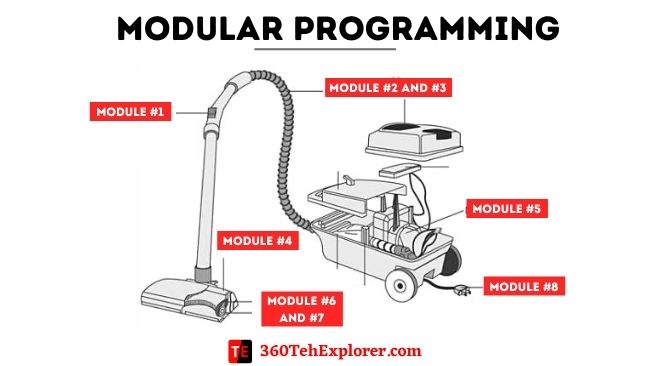
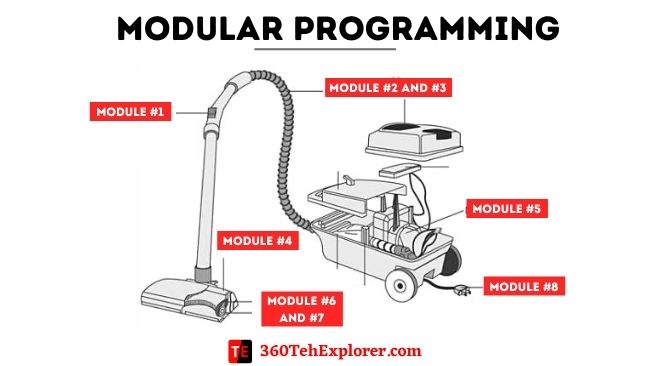
Thnk this vacuum cleaner is your software and its part are the modules.
Modular programming is not a new approach it is here from the 1960s, but its use and one of the most used approach to software development.
Modular programming is closely related to “structured programming” and “OOPs”, all having the same goal of better maintainability of large software programs and systems by decomposition into smaller pieces, and all originating around the 1960s.
Advantages of modular programming
Here are some of the major advantages or benefits of modular programming.
These are the main reason why other (and me) recommend you to have modular programming is a good choice.
- Reusable Code
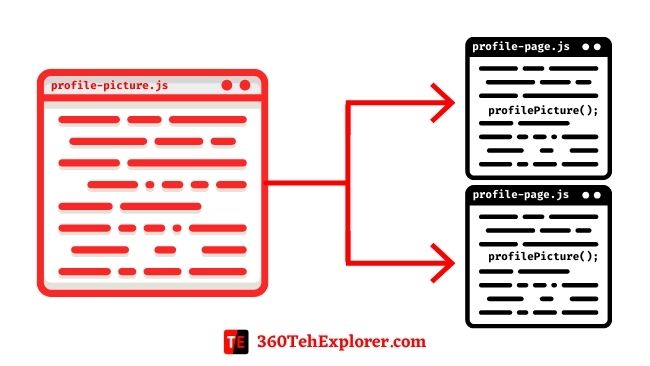
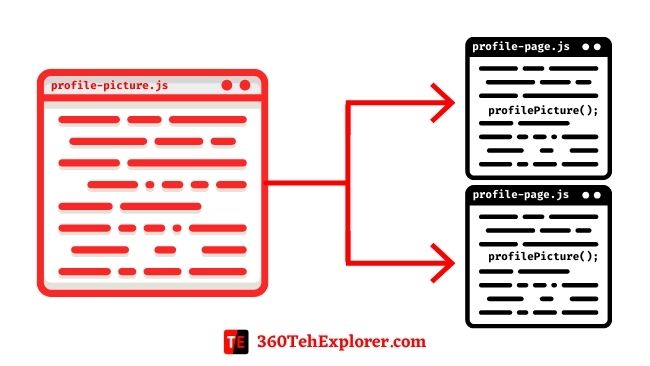
Divide a large software into multiple independent modules and make each class/method do just one specific task. Modularization makes code easy to understand and more maintainable. It allows easy reuse of methods or functions in a program and reduces the need to write repetitively.
- Ease of Maintenance
Having a program broken into smaller sub-programs allows for easier management. The separate modules are easier to test, implement, or design. These individual modules can then be used to develop the whole program.
- Easier to Debug
Debugging is one of the major parts of programming, and also one of the most irritating parts of development, When debugging large programs, how and when any bugs occur can become a mystery. This can take much of your valuable time as he searches through lines and lines of code to find out where an error occurred, however, then each discrete task has its discrete section of code. So, if there is a problem in a particular function, the programmer knows where to look and can manage a smaller portion of the code.
- Collaborate
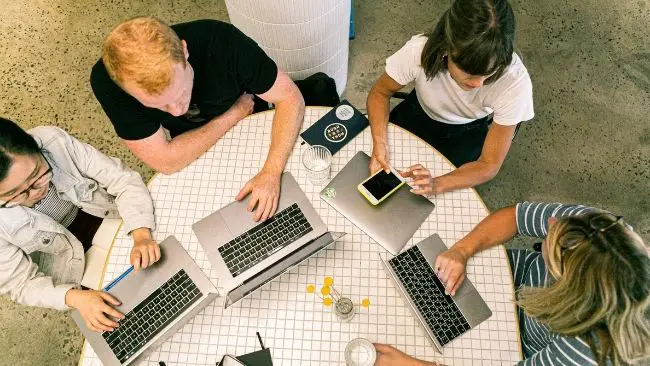
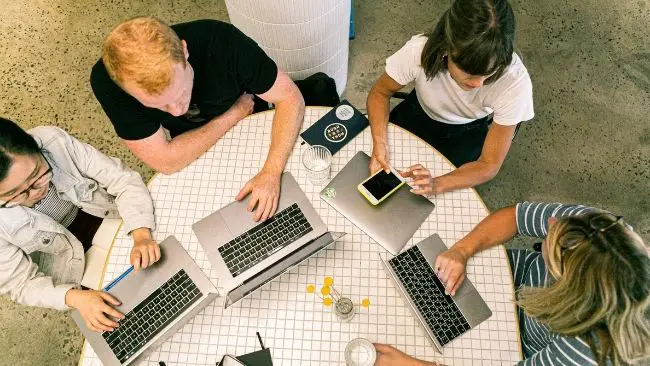
Another advantage of this modular approach is that it allows for collaborative programming. Instead of giving a large job to a single programmer, you can split it up into a large team of programmers. Each programmer can be given a specific task to complete as part of the overall software application. Then, in the end, all of the various work from the programmers is compiled to create the program. This helps speed up the work.
Disadvantages of modular programming
- This can lead to problems with variable names
When a program is divided into smaller modules, each module has its own set of variables. However, this can cause confusion if variable names are not clear and consistent across the entire program. For instance, different modules using the same variable name to refer to different things or inconsistent variable naming across modules can lead to errors and confusion. It is also easy to mistakenly reuse a variable name in another module, leading to unintended consequences. Therefore, it is crucial to ensure that variable names are clearly defined and consistently used across all modules to prevent confusion and errors in the program.
- This can lead to problems when modules are linked because the link must thoroughly be tested
Modular programming has a potential downside when modules are linked together. This is because it is crucial to test the linking process thoroughly to ensure that the modules work seamlessly with each other. If there are errors or bugs in one module, it can affect the functionality of other linked modules, leading to difficulties in fixing the issues. Therefore, it is vital to conduct a thorough testing of the linking process to avoid any such problems and ensure that the program functions smoothly.
Languages that support modular programming
Programming Languages that support the module programming concept:
- C++
- C#
- Dart
- Go
- Java
- Objective-C
- Ruby
- JavaScript
Ada, Algol, BlitzMax, Clojure, COBOL, Common_Lisp, D, C, Erlang, Elixir, Elm, F, F#, Fortran, Go, Haskell, IBM/360 Assembler, Control Language (CL), IBM RPG, MATLAB, ML, Modula, Modula-2, Modula-3, Morpho, NEWP, Oberon, Oberon-2, OCaml, several derivatives of Pascal (Component Pascal, Object Pascal, Turbo Pascal, UCSD Pascal), Perl, PL/I, PureBasic, Python, R, Rust, Visual Basic .NET, and WebDNA.
Note: There are some other programming languages as well.
Modular programming in Python
In Python modular approach is already widely used for example: when you import a package in Python you are using the modular approach.
Here is a code sample of this:
import math, random
You can also create a custom module for your software application.
Here is a code sample of this:
Create a module in Python
Follow this step-by-step guide to create a module in Python:
- Create an ‘example.py’ file and paste the below code.
# ---- example.py -----
# Python Module example
def add(a, b):
"""This program adds two
numbers and return the result"""
result = a + b
return result
- Import your module.
import example
This not enough for large software there are other things to learn in modular programming in Python.
Modular programming in Java
Modules are normally written as independent functions (called methods in Java).
Here is a code sample of this:
static int addition(int x,int y)
{
return x+y;
}
And use it as:
public static void main(String[] arg)
{
int a,b,c;
Scanner sc=new Scanner(System.in);
System.out.println("Enter first number");
a=sc.nextInt();
System.out.println("Enter second number");
b=sc.nextInt();
c=addition(a,b);
System.out.println(" Addition of two numbers is : "+c);
}
Java is not only made up of methods. It is an object-oriented programming language where functionality is broken down into objects. An object is implemented as a Java class and has a name, some characteristics (called attributes), and some actions (methods) that can be performed on it or by it.
This not enough for large software there are other things to learn in modular programming in Java.
Modular programming in C++
The smallest unit of code in C++ is called a function.
Putting functions or a group of functions into a separate module (source file) is modular programming in C++.
Here is a code sample of this:
//function definition
int addition(int a,int b)
{
return (a+b);
}
And use it as:
int main()
{
int num1; //to store first number
int num2; //to store second number
int add; //to store addition
//read numbers
cout<<"Enter first number: ";
cin>>num1;
cout<<"Enter second number: ";
cin>>num2;
//call function
add=addition(num1,num2);
//print addition
cout<<"Addition is: "<<add<<endl;
return 0;
}
This not enough for large software there are other things to learn in modular programming in C++.
General Modular programming terms.
Function
a function is a block of code that performs a specific task. It is like a sub-program within the main program that can be called from different parts of the program. Functions can take input arguments, process them, and return output values. They help break down the program into smaller, more manageable tasks, making it easier to understand and maintain.
What modules are called in many predominant programming languages of today?
Function call
A function’s using or invoking another function.
A function call is a way of invoking a function from another part of the program. It is like making a request to the sub-program to perform a specific task. When a function is called, the program execution jumps to the function code, executes it, and then returns to the calling code. Function calls help break down a program into smaller, more manageable tasks, making it easier to write, understand, and maintain.
Function definition
The code defines what a function does.
A function definition is a block of code that specifies what the function does when it is called. It is like a blueprint or recipe for the function, describing the input arguments it takes, the processing it performs, and the output values it returns. Function definitions help break down a program into smaller, more manageable tasks, making it easier to write, understand, and maintain. They are usually placed at the beginning of the program and can be called from different parts of the program as needed.
Function prototype
A function’s communications declaration to a compiler.
A function prototype is a declaration of a function that specifies its name, input arguments, and return type. It is like a preview or trailer for the function, giving the compiler information about the function before it is defined in the program. Function prototypes help to ensure that functions are called with the correct number and types of arguments and that the return value is used properly. They are usually placed at the beginning of the program before the function definitions and help to organize and streamline the code.
Identifier name
The name was given by the programmer to identify a function or other program items such as variables.
A identifier name is a label given to a variable, function or another program element to identify and refer to it in the code. It is like a name tag that helps to distinguish one element from another. Identifier names should be meaningful, clear, and concise, reflecting the purpose and function of the element they represent. It is important to choose identifier names carefully to avoid confusion and errors in the program. Consistent and descriptive identifier names make the program easier to read, understand, and maintain.
Modularization
The ability to group some lines of code into a unit that can be included in our program.
modularization is the process of breaking down a program into smaller, more manageable modules or functions. It is like dividing a complex task into smaller, more manageable sub-tasks. Modularization helps to organize the code, making it easier to write, understand, and maintain. It allows different parts of the program to be developed independently and tested separately, reducing the risk of errors and bugs. Modularization also facilitates code reuse, as modules can be used in different programs or combined to create new functionalities.