This time we will deal with a programming language that is very easy on some sides but difficult on others. Well, I am talking about C ++ (or C plus plus).
C++ is the language I highly recommend for competitive programming
C++ gives complete control over the hardware to the coders which is awesome but difficult for beginners.
So in this article, I am going to explain some of the basics of C++.
What is C++
C++ is a high-language general-purpose programming language, C ++ derives from C by Bjarne Stroustrup at Bell Labs circa 1980. C++ is very similar to C (invented by Dennis Ritchie). It was developed as a cross-platform improvement of C to provide developers with a higher degree of control over memory and system.
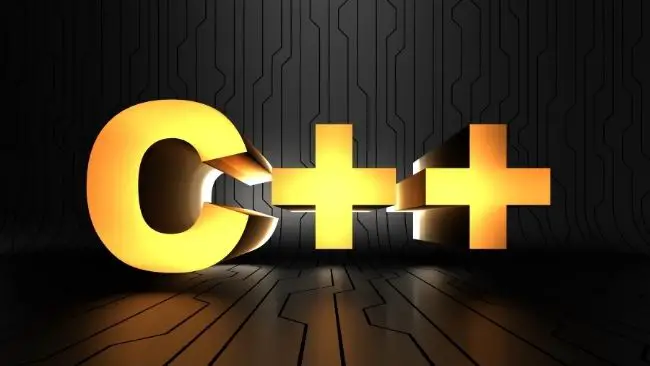
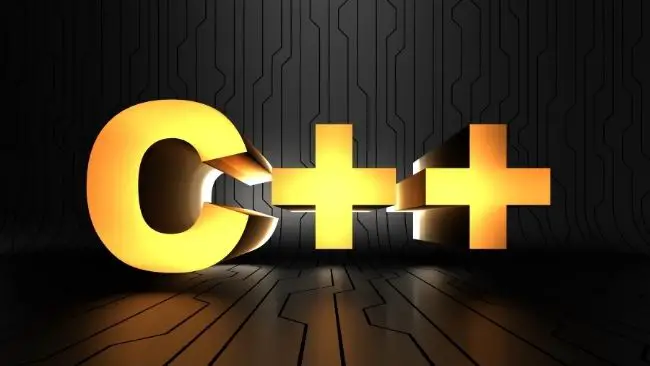
Let’s talk more specifically now, what is special about C ++ that other compilers don’t have? It simply has similarities in some respects with many languages such as PHP and Java, but it is much more simplified being a high-level language and not a low-level language.
In C ++ the lines of code we encounter for sure are:
int Main | Main of the code |
cin >> | Input of a variable from the keyboard |
cout << | Output of variable |
return 0 | Close the program |
Advantages of C++
- The introduction of the object-oriented programming paradigm,
- Virtual functions,
- Operator overloading
- Multiple inheritances,
- Template and exception handling.
Basics of C++
1. C++ Keywords
The following table shows the reserved words/keywords in C++. These reserved words may not be used as constant or variable or any other identifier names.
asm | else | new | this |
auto | enum | operator | throw |
bool | cprivate | true | false |
break | export | protected | try |
case | extern | public | typedef |
char | float | reinterpret_cast | typename |
class | for | return | union |
const | friend | short | unsigned |
const_cast | goto | signed | using |
default | inline | static | void |
delete | int | static_cast | volatile |
do | long | struct | wchar_t |
double | mutable | switch | while |
dynamic_cast | namespace | template | typeid |
catch | false | register |
2. Whitespace and comments in C++
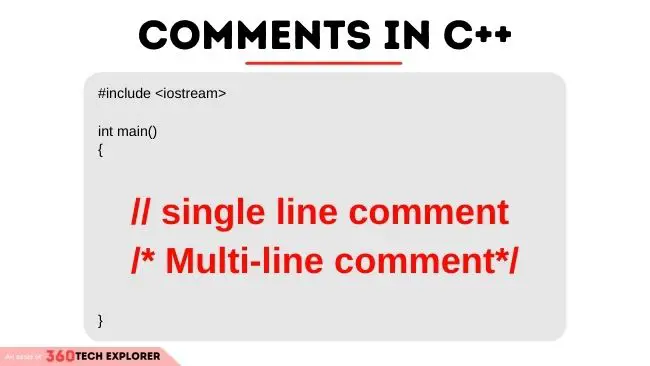
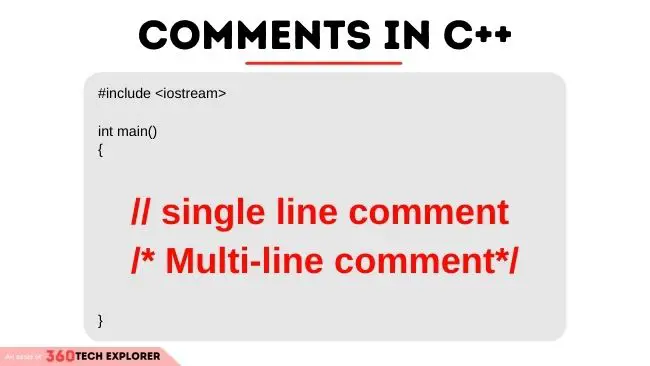
A line containing only whitespace, possibly with a comment, is known as a blank line, and the C++ compiler ignores it.
Whitespace is the term used in C++ to describe blanks, tabs, newline characters, and comments. Whitespace separates one part of a statement from another and enables the compiler to identify where one element in a statement, such as an int, ends and the next element begins.
Statement 1:
int age;
In the above statement, there must be at least one whitespace character (usually space) between int and age for the compiler to be able to distinguish them.
Statement 2:
fruit = apples + oranges; // Get the total fruit
In the above statement 2, no whitespace characters are necessary between fruit and =, or between = and apples, although you are free to include some if you wish for readability purposes.
3. C++ Datatypes
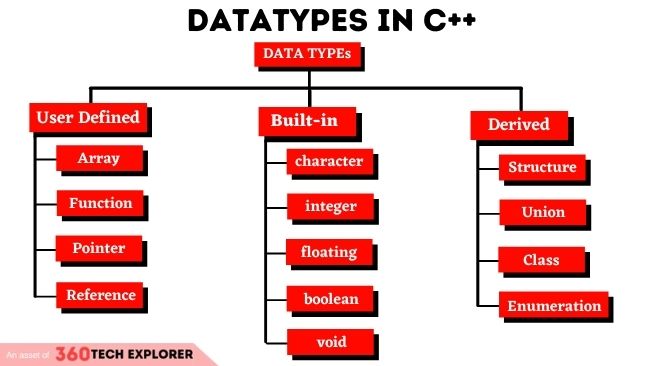
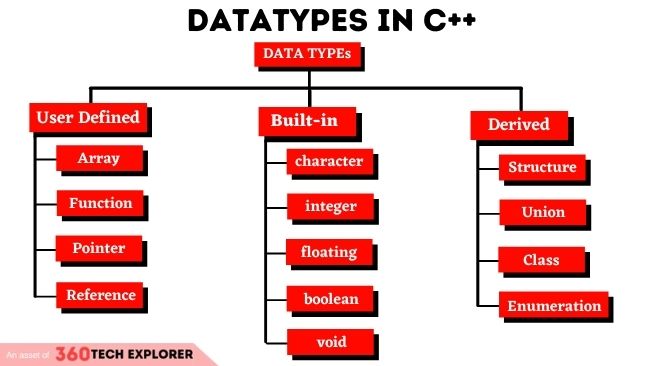
Each programming language defines formats for representing data. C ++ is a language characterized by static typing: this means that each variable must be associated with a type.
Since everything is reduced to a sequence of bits during the execution of a program, there must be a mechanism that allows us to correctly interpret the meaning of these sequences and define a set of operations on them.
The definition of types solves this problem and allows us to operate on different types of data.
Primitive or fundamental types
Primitive or fundamental types are those that allow you to represent “simple” information, such as a single numeric value, text, Boolean values, etc.
Each type definition has the following properties:
- the size, expressed in the number of bits necessary for the representation of a variable;
- the range of representing able values;
- the distribution of representing able values;
- In the case of numeric types, the sign.
To some types, in particular the numeric ones, modifiers can be applied that alter their size and sign with respect to the predefined characteristics of the type. As we shall see, these modifications correspond to a variation of the range of representable values.
- Whole numbers
The int type is used to represent natural numbers or relative integers. It is possible to alter the size and/or the sign of the type int by using the appropriate modifiers short, long, long long, signed, and unsigned.
The minimum size of the integer type (with or without modifiers) is defined by the C ++ standard. The actual size depends on the data model being used. The latter is simply the convention used to give types a fixed size depending on the processor architecture (x86 or x64) and the operating system.
Data models are commonly identified by an acronym consisting of the symbols I (integer), L (long), P (pointer), and LL (long long), followed by the number of bits used for the representation (32 or 64). For example, ILP32 identifies the data model where integers, longs, and pointers have size 32. The following table shows the type size int for the most common data models.
Guy Standard C ++ ILP32 LLP64 ILP64
(signed | unsigned) short int min. 16 bit 16 bit 16 bit 16 bit
(signed | unsigned) int min. 16 bit 16 bit 32 bit 32 bit
(signed | unsigned) long int min. 32 bit 32 bit 32 bit 64 bit
(signed | unsigned) long long int min. 64 bit 64 bit 64 bit 64 bit
- Characters
The char type is used for the representation of single characters or text strings. CharSign modifiers can be used for the type, but not the size modifiers, which are traditionally 8 bits, sufficient for ASCII encoding.
For different encodings, such as UTF, there are types wchar_t (wide char), char16_t, and char32_t. The table Given below will show the main features.
Type | Standard C ++ | ILP32 | LLP64 | ILP64 |
---|---|---|---|---|
(signed | unsigned) short int | min. 16 bit | 16 bit | 16 bit | 16 bit |
(signed | unsigned) int | min. 16 bit | 16 bit | 32 bit | 32 bit |
(signed | unsigned) long int | min. 32 bit | 32 bit | 32 bit | 64 bit |
(signed | unsigned) long long int | min. 64 bit | 64 bit | 64 bit | 64 bit |
- Boolean values
The bool type is used to represent two values: true (true) and false (false). The size is typically 8 bits.
- Void
The void type is used to indicate an empty or undefined set of values. It is an incomplete type, in the sense that it cannot be allocated; references or arrays of void elements cannot be used. It is actually only definable as a pointer or as a return type for functions that do not return a result.
Dimensions and limits of the fundamental types
Although the bit size of fundamental types is specified by the standard, there are special exceptions or interpretations. In the case of uncertainties we can resort to two tools that the language makes available to us:
The size of (type) operator;
std: numeric_limits.
The following listing produces a table with the size in bytes and the maximum and minimum values of many fundamental types. Here I deliberately omit the detailed explanation line by line and focus only on the result produced.
#include <iostream>
#include <limits>
#include <iomanip>
using namespace std;
int main()
{
int w = 20;
cout << setw(w) << "TIPO"<< setw(w) << "BYTES"<< setw(w) << "MIN"<< setw(w) << "MAX" << endl;
cout << setw(w) << "int"<< setw(w) << sizeof(int)<< setw(w) << numeric_limits<int>::min()<< setw(w) << numeric_limits<int>::max() << endl;
cout << setw(w) << "char"<< setw(w) << sizeof(char)<< setw(w) << (int) numeric_limits<char>::min()<< setw(w) << (int) numeric_limits<char>::max() << endl;
cout << setw(w) << "float"<< setw(w) << sizeof(float)<< setw(w) << numeric_limits<float>::min()<< setw(w) << numeric_limits<float>::max() << endl;
cout << setw(w) << "double"<< setw(w) << sizeof(double)<< setw(w) << numeric_limits<double>::min()<< setw(w) << numeric_limits<double>::max() << endl;
cout << setw(w) << "bool"<< setw(w) << sizeof(bool)<< setw(w) << numeric_limits<bool>::min()<< setw(w) << numeric_limits<bool>::max() << endl;
/* Attenzione: queste righe generano errori di compilazione!
cout << setw(w) << "void"
<< setw(w) << sizeof(void)
<< setw(w) << numeric_limits<void>::min()
<< setw(w) << numeric_limits<void>::max() << endl;
*/
return 0;
}
The compound types
Compound types are those formed by aggregation from fundamental types. These types are typically defined with the help of struct or class and template constructs, which I will learn more about later.
The C ++ standard incorporates not only the syntactic characteristics of the language but also the definition of some libraries, the implementation of which is then left to the individual platforms.
One of these is the String library, which includes the definition of the string type.
- Strings
The string type allows you to manipulate sequences of characters more easily than handling an array of chars. This is possible thanks to the definition of some methods that allow us to establish the size of the string, concatenate different strings, search and/or replace character sequences.
Since the typed string is a compound type, it is based on the definition of primitive types, and in particular the fonts. There are numerous variations of the typed string, depending on the underlying basic type:
- std:string( char)
- std:wstring( wchar_t)
- std:u16string( char16_t)
- std:u32string( char32_t)
The most frequently used is std: string. For the use of the string library, it is necessary to include the relative header file with the following instruction in your sources.
#include <string>