Programming doesn’t mean solving problems programming means solving the problems in the right way.
Any fool can write code that a computer can understand. Good programmers write code that humans can understand.
― Martin Fowler
What is Programming Paradigm
While solving problems, the way you structure your program is called a programming paradigm. The programming paradigm is not dependent on the programming language. The most popular programming paradigm is OOPs but the best programming paradigm is that which reduces the complicity of the understanding and debugging process.
Put simply,
Programming Paradigm is a way or style of your program.
List of Programming Paradigms
Tons of programming paradigms were developed and continually evolving.
Here I mention almost all of the programming paradigms.
- Object-oriented programming (OOPs)
- Functional programming
- Procedural programming
- Imperative programming
- Declarative programming
- Logic programming
- Structured programming
- Event-driven programming
- Modular programming
- Aspect-oriented programming
- Prototype-based programming
- Data-driven programming
Programming Paradigms
Object-oriented programming (OOPs)
OOPs is the most common term in programming. This is the most common paradigm.
What is Object-oriented programming?


Object-Oriented programming system (OOPs) is a programming paradigm that is based on the concept of classes and objects. It is used to structure a complex program into simple, reusable blocks of code classes, which are used to create individual instances of objects.
Example (in C++):
class MyClass { // The class
public: // Access specifier
int myNum; // Attribute (int variable)
string myString; // Attribute (string variable)
};
int main() {
MyClass myObj; // Create an object of MyClass
// Access attributes and set values
myObj.myNum = 15;
myObj.myString = "Some text";
// Print attribute values
cout << myObj.myNum << "n";
cout << myObj.myString;
return 0;
}
Languages that support:
- Java
- JavaScript
- Python
- C++
- Visual Basic .NET
- Ruby
- Scala
- PHP
Functional programming
In recent years, functional programming has become so popular because of Javascript.
What is Functional programming?
Functional programming is a programming paradigm where programs are constructed by applying and composing functions, avoiding shared state, mutable data, and side effects. Functions mode of data and methods, methods can modify the value of data itself.
Example in Javascript:
function isPrime(number){
for(let i=2; i<=Math.floor(Math.sqrt(number)); i++){
if(number % i == 0 ){
return false;
}
}
return true;
}
isPrime(15); //returns false
Languages that support:
- JavaScript
- Haskell
- OCaml
- Scala
- Clojure
- Racket
Procedural programming
Procedural programming (which is also imperative) splitting those instructions into procedures.
What is Procedural programming?
Procedural programming is a programming paradigm that is derived from imperative programming. This paradigm uses a linear top-down approach and treats data and procedures as two different entities, based on the concept of the procedure call. Procedures simply contain a series of computational steps to be carried out.
Example in C:
#include <stdio.h>
int main()
{
int sum = 0;
int i =0;
for(i=1;i<11;i++){
sum += i;
}
printf("The sum is: %dn", sum); //prints-> The sum is 55
return 0;
}
Languages that support:
- C
- Java
- C++
- Pascal
- ColdFusion
Imperative programming
Imperative programming (from the Latin word, imperare = command) is the oldest programming paradigm. A program based on this paradigm is made up of a clearly defined sequence of instructions to a computer.
What is Imperative programming?
Imperative programming is a programming paradigm that uses commends or statements that change a program’s state. In much the same way that the imperative mood in natural languages expresses commands, an imperative program consists of commands for the computer to perform.
Example in C:
int main()
{
int sum = 0;
sum += 1;
sum += 2;
sum += 3;
sum += 4;
sum += 5;
sum += 6;
sum += 7;
sum += 8;
sum += 9;
sum += 10;
printf("The sum is: %dn", sum); //prints-> The sum is 55
return 0;
}
Languages that support:
- C
- C#
- C++
- Python
- Fortran
- Java
- Pascal
- ALGOL
- Assembler
- BASIC
- COBOL
- Ruby
Declarative programming
Declarative programming is a style of building programs that express the logic of a computation without talking about its control flow.
What is declarative programming?
Declarative programming is a programming paradigm. The declarative property is where there can exist only one possible set of statements that can express each specific modular semantic. The imperative property3 is dual, where semantics are inconsistent under composition and/or can be expressed with variations of sets of statements.
Example in SQL:
SELECT * FROM Users WHERE Country='Mexico';
Languages that support:
- SQL
- Haskell
- Prolog
- Lisp
- Miranda
- Erlang
Logic programming (Constraint)
The logic programming paradigm is based on formal logic.
What is logic programming?
Logic programming is a programming paradigm where program statements express facts and rules about problems within a system of formal logic. Rules are written as logical clauses with a head and a body; for instance, “H is true if B1, B2, and B3 are true.” Facts are expressed similar to rules, but without a body; for instance, “H is true.”
Example:
/*We're defining family tree facts*/
father(John, Bill).
father(John, Lisa).
mother(Mary, Bill).
mother(Mary, Lisa).
/*We'll ask questions to Prolog*/
?- mother(X, Bill).
X = Mary
Languages that support:
- Absys
- ALF (algebraic logic functional programming language).
- Algorithmic program debugging
- ASP (Answer Set Programming)
- Logtalk
- Parlog
- Planner
- PROGOL
- Prolog
- Prolog++
- Prova
- .QL
Structured programming
What is structured programming?
Structured programming is a programming paradigm that makes extensive use of the structured control flow constructs of selection (if/then/else) and repetition (for and while), block structures, and subroutines in contrast to using simple tests and jumps such as the go to statement, which can lead to “spaghetti code” that is potentially difficult to follow and maintain.
Example in BASIC:
DIM I
FOR I = 0 TO 9
PRINT "HELLO"
NEXT
Languages that support:
- C
- C++
- C#
- BASIC
- PHP
- Ruby
- PERL
- ALGOL
- Pascal
- PL/I
- Ada
Event-driven programming
What is event-driven programming?
Event-driven programming is a programming paradigm in which the flow of program execution is determined by events – for example, user action such as a mouse click from the OS or another program. An event-driven application is designed to detect events as they occur and then deal with them using an appropriate event-handling procedure.
Example in Python:
import turtle
turtle.setup(400,500) # Determine the window size
wn = turtle.Screen() # Get a reference to the window
wn.title("Handling keypresses!") # Change the window title
wn.bgcolor("lightgreen") # Set the background color
tess = turtle.Turtle() # Create our favorite turtle
# The next four functions are our "event handlers".
def h1():
tess.forward(30)
def h2():
tess.left(45)
def h3():
tess.right(45)
def h4():
wn.bye() # Close down the turtle window
# These lines "wire up" keypresses to the handlers we've defined.
wn.onkey(h1, "Up")
wn.onkey(h2, "Left")
wn.onkey(h3, "Right")
wn.onkey(h4, "q")
# Now we need to tell the window to start listening for events,
# If any of the keys that we're monitoring is pressed, its
# handler will be called.
wn.listen()
wn.mainloop()
Languages that support:
- Python
- Javascript
- C++
- Java
- VB
- .NET
All object-oriented and visual languages support event-driven programming.
Modular programming
What is modular programming?
We have a separate article about modular programming that you might like: click here to view.
Example:
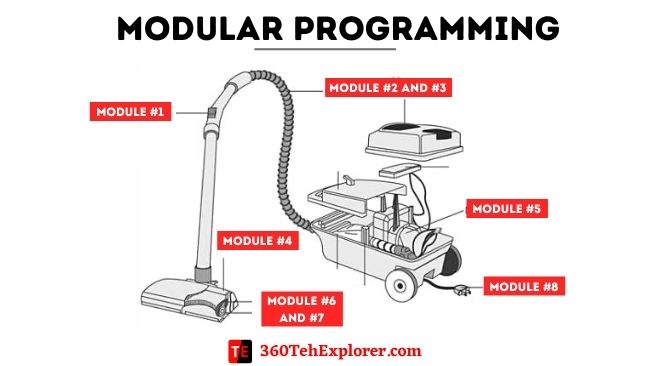
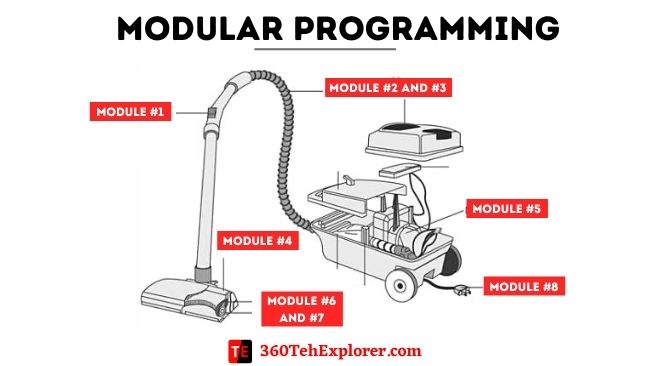
Languages that support:
- Javascript
- Python
- Java
- Go
- C++
- C#
- Dart
- Ruby
Almost all high-level lanuages
Aspect-oriented programming
What is Aspect-oriented programming?
Aspect-oriented programming is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. It does by adding behavior to existing code without modifying the code itself, instead separately specifying which code is modified via a “pointcut” specification.
Example in Java:
void transfer(Account fromAcc, Account toAcc, int amount, User user,
Logger logger, Database database) throws Exception {
logger.info("Transferring money...");
if (!isUserAuthorised(user, fromAcc)) {
logger.info("User has no permission.");
throw new UnauthorisedUserException();
}
if (fromAcc.getBalance() < amount) {
logger.info("Insufficient funds.");
throw new InsufficientFundsException();
}
fromAcc.withdraw(amount);
toAcc.deposit(amount);
database.commitChanges(); // Atomic operation.
logger.info("Transaction successful.");
}
Languages that support:
- .NET Framework languages
- C / C++
- Groovy
- Haskell
- Java
- AspectJ
- JavaScript
- Lua
- Perl
- PHP
- Python
- Ruby
- XML
Prototype-based programming
Prototype-based programming is a style of programming in which already-created objects are cloned and reused to construct a program or applications.
What is prototype-based programming?
Prototype-based programming is a programming paradigm. Prototype-based programming is a style of object-oriented programming in which classes are not explicitly defined, but rather derived by adding properties and methods to an instance of another class or, less frequently, adding them to an empty object.
Example in Javascript:
var a = {a: 1};
// a ---> Object.prototype ---> null
var b = Object.create(a);
// b ---> a ---> Object.prototype ---> null
console.log(b.a); // 1 (inherited)
var c = Object.create(b);
// c ---> b ---> a ---> Object.prototype ---> null
var d = Object.create(null);
// d ---> null
console.log(d.hasOwnProperty);
// undefined, because d doesn't inherit from Object.prototype
// Base Object always has hasOwnProperty
Languages that support:
- Javascript
- Python
- Ruby
- Typescript
- Lua
Data-driven programming
What is Data-driven programming?
Data-driven programming is a programming paradigm where the data itself controls the flow of the program and not the program logic. It is a model where you control the flow by offering different data sets to the program where the program logic is some generic form of flow or of state changes.
Example:
from User import User
from EmailService import EmailService
#
# rules data
#
rule_set = [
{
"country": ["fr"],
"subject": "Bonjour et bienvenue sur notre site",
"content": "Bonjour et Bienvenue sur notre tout nouveau site. Contactez l'équipe de support si vous avez des..."
},
{
"country": ["us", "ca"],
"subject": "Welcome to our site",
"content": "Hello and Welcome to our brand new site. Contact support team if you have any questions."
},
{
"min_age": 18,
"subject": "Important reminder",
"content": "Important: Please note that user under the age of 18 must provide a signed waiver from a parent ..."
},
{
"payment_status": "Unverified",
"subject": "Reminder about your payment status",
"content": "Please don't forget to update your payment information on our site"
}
]
#
# main function
#
def main():
#
# Fetch the current user
#
user = User()
user.load_from_session()
#
# Process the config data and fire actions
#
for rule in rule_set:
# Process country rule
if "country" in rule and user.country in rule["country"]:
EmailService.send(user.email, rule["subject"], rule["content"])
# Process minimum age rule
if "min_age" in rule and user.age < rule["min_age"]:
EmailService.send(user.email, rule["subject"], rule["content"])
# Process payment status rule
if "payment_status" in rule and user.payment_status == rule["payment_status"]:
EmailService.send(user.email, rule["subject"], rule["content"])
#
# run main
#
if __name__ == "__main__":
main()
Languages that support:
- Python
- Oz
- Perl
- sed
- Lua
- Clojure
- fdm
- maildrop
- procmail
- Sieve
Conclusion
Programming paradigms reduce the complexity of programs. Programmers must know the programming paradigm approach. Each programming paradigm has its advantages and disadvantages.
Please feel free to let me know if you have any questions.
Thank you for reading.